Exploring different coding paradigms is an interesting and demanding path for a backend-heavy software developer.
Coming from the generation that wrote procedural codes and then transitioning into Object Oriented Programming (OOP) when I started working with organised teams.
It was a different ballgame requiring discipline and adhering to programming standards.
This article is a compilation of my experiences, lessons, and knowledge I gathered about;
- the importance of coding standards
- class organisation
- frameworks and
- the seamless migration of legacy codebases.
Note that the examples I will give in this article are typically PHP-based, but the same principles apply to other OOP-focused programming languages like JavaScript, TypeScript, Java, Python, etc.
Procedural Programming Phase (Spaghetti Coding)
Procedural programming was the beginning of my journey into software development. This paradigm is usually simple when developing very little pieces of code snippets.
However, in more complex requirements, those snippets are grouped into separate files and included in the runtime when required or preferably into separate functions.
This method indisputably helped me accomplish tasks, but over time I realised the limitations of this method when it comes to developing more complicated applications.
These limitations include;
- Having different files that accomplish similar requirements,
- complexity in managing codebase and unorganised workflows—which increases as the application grows.
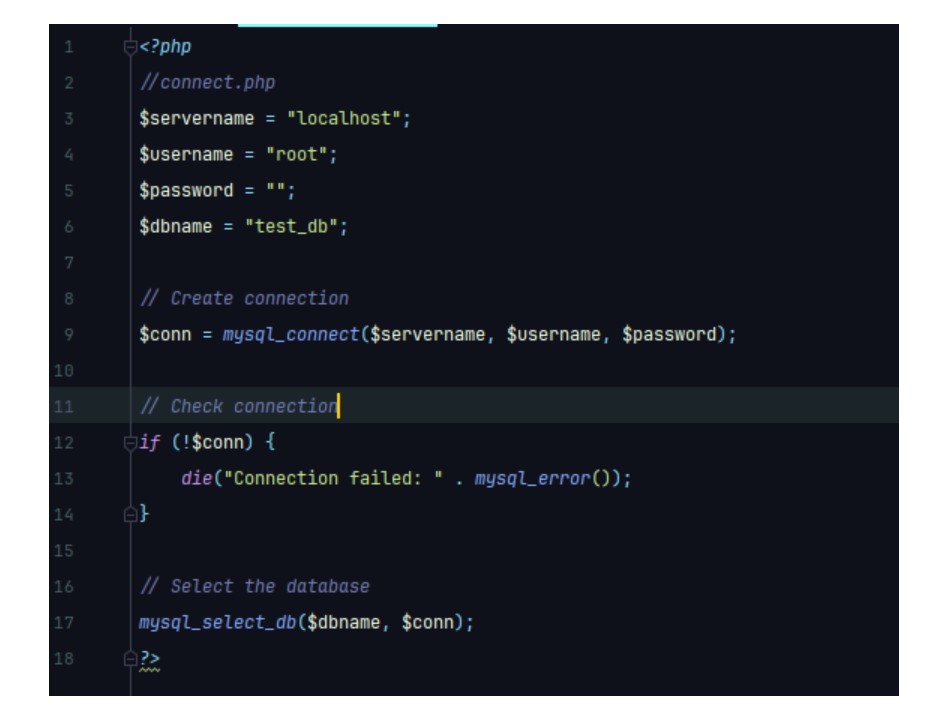
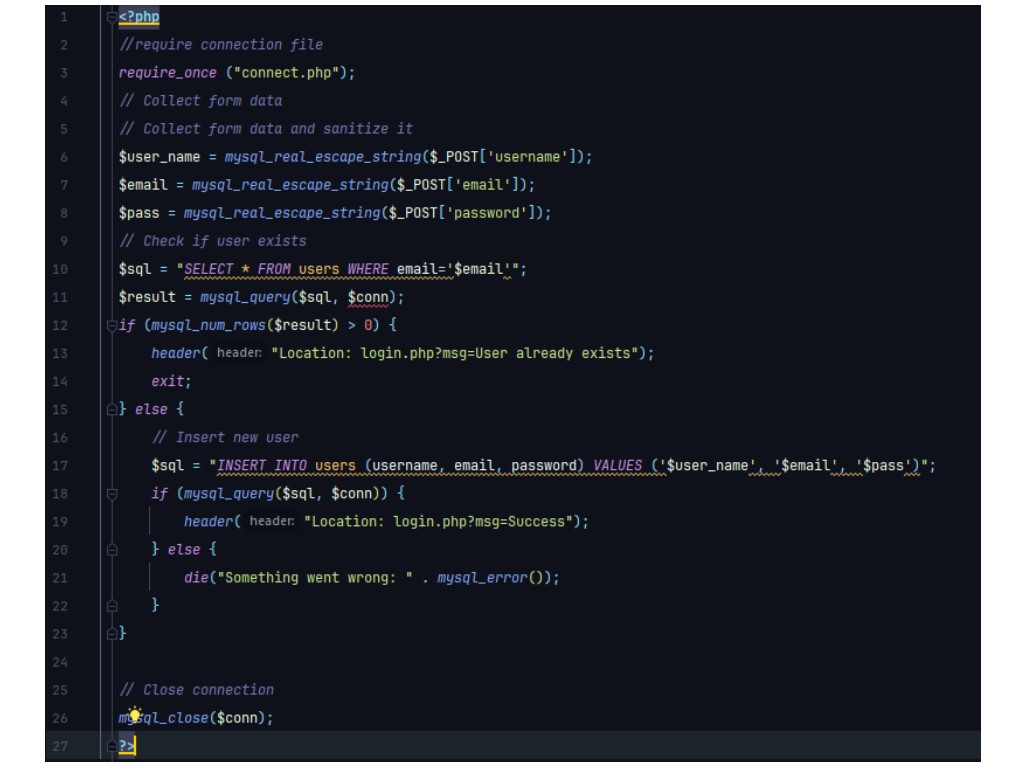
The above code snippet will work for a simple user registration page but will become more complex when adding extra features such as email verifications, etc.
Transition into Object-Oriented Programming
I couldn’t wave off the idea of data and action encapsulation within objects as it seemed to be the solution to my ever-increasing project complexity.
This idea prompted my first transition into object-oriented programming (OOP).
My early attempts at object-oriented programming were filled with setbacks resulting from my ignorance of fundamental coding concepts.
The classes I took, though rigid, turned out incompetent as they excluded several fundamental principles of effective design.
My Discovery: The Need for Coding Standard
My understanding of the significance of coding standards was fine-tuned when I began collaborating with well-structured teams.
Guidelines like SOLID (Single Responsibility, Open-Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion) were followed by these groups.
These principles are more than theoretical exercises; they provide actual instructions for more resilient, scalable, and easy-to-maintain codes.
There are numerous things to understand about programming principles but I will explain some important ones.
1. Single Responsibility Principles (SRP)
This is one of the first principles I embraced.
Depending on your preferred programming language, the main requirement for this principle is knowledge of Object Oriented Programming, how it works, and how to organise your codes into classes and methods.
The Single Responsibility Principle (SRP) states that a class should have only one reason to change.
I started implementing SRP by breaking down my monolithic classes into smaller and precise ones. This made my codebase easier to understand and maintain.
This saved me the cost of breaking my functionalities into separate files and including them when needed.
Now let’s apply what we’ve learned so far. We will convert the previous functionalities into classes.
If we are applying the SRP;
- We will have a class called Database, which will be in charge of making connections to the database.
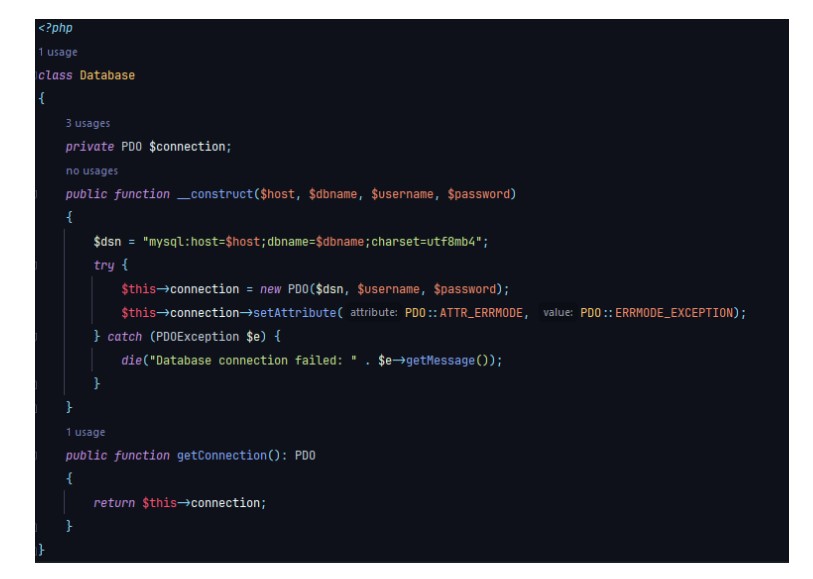
2. We will also define another class called AuthController which will house all the “Methods” that control the Authorization such as register, login, verifyEmail, etc.
2. SOLID Principle: Other Components of SOLID Principles.
My coding techniques were further refined as I continued to adhere to the remaining SOLID principles.
My understanding of the importance of making sure that subclasses and their base classes are compatible came from—the Liskov Substitution Principle (LSP).
I was inspired to build more detailed and precise interfaces by the—Interface Segregation Principle (ISP), and finally was able to separate high-level modules from low-level implementations by the–Dependency Inversion Principle (DIP).
Programming Frameworks
I started leveraging programming frameworks as I was learning coding standards. Here is why;
By providing a standardised basis for application development, frameworks guarantee consistency and best practices.
I was able to reduce the amount of boilerplate code I needed to write because they also offered various built-in functionalities.
Other Benefits of Using Frameworks
I was also able to drastically improve my coding skills after adopting frameworks like React(which relies on JavaScript) for front-end development and Laravel(which relies on PHP) for back-end development.
By enforcing coding standards and architectural principles, these frameworks strengthened and simplified the maintenance of my apps.
During my learning phase, the community support and detailed documentation were also beneficial.
Migrating Legacy Codebases
This migration was another leap in my programming journey.
From the knowledge I gathered to this point, I knew it was time to transform some of my legacy codebases.
Transitioning from an OOP codebase that was procedural and ill-structured to one that followed conventional coding techniques was one of the most satisfying tasks I ever encountered. A slow, iterative strategy was crucial for a smooth migration.
Firstly before I started reworking, I examined my legacy codebases and ensured I followed coding standards for the most important parts of the code.
I was able to thoroughly test each modification before going on to the next by refactoring it in small steps.
Using the Single Responsibility Principle, I divided huge classes into more manageable ones. Subsequently, I made sure my classes could be extended without any changes by applying the Open-Closed Principle.
As I refactored my code, I noticed significant performance improvements and my applications became more efficient because I organised my classes better and reduced dependencies.
The use of design patterns also contributed to these optimizations, providing tried-and-tested solutions to common problems.
Conclusion
My transition from procedural to a standard codebase has been a life-changing experience for me.
My adoption of coding standards like the SOLID principles and the use of frameworks transformed me into a more disciplined and efficient developer.
The process of transferring historical codebases has been incredibly gratifying, despite its challenges, as it has influenced the creation of maintainable and streamlined applications.
Generally, this series of experiences revealed the trend in the software development industry. It is always changing.
So, it’s important to adapt to change, learn best practices, and follow coding standards to keep up with the trend. Beyond this, we can also build long-lasting apps that are scalable, stable, and easy to maintain if we learn and adopt these standards.